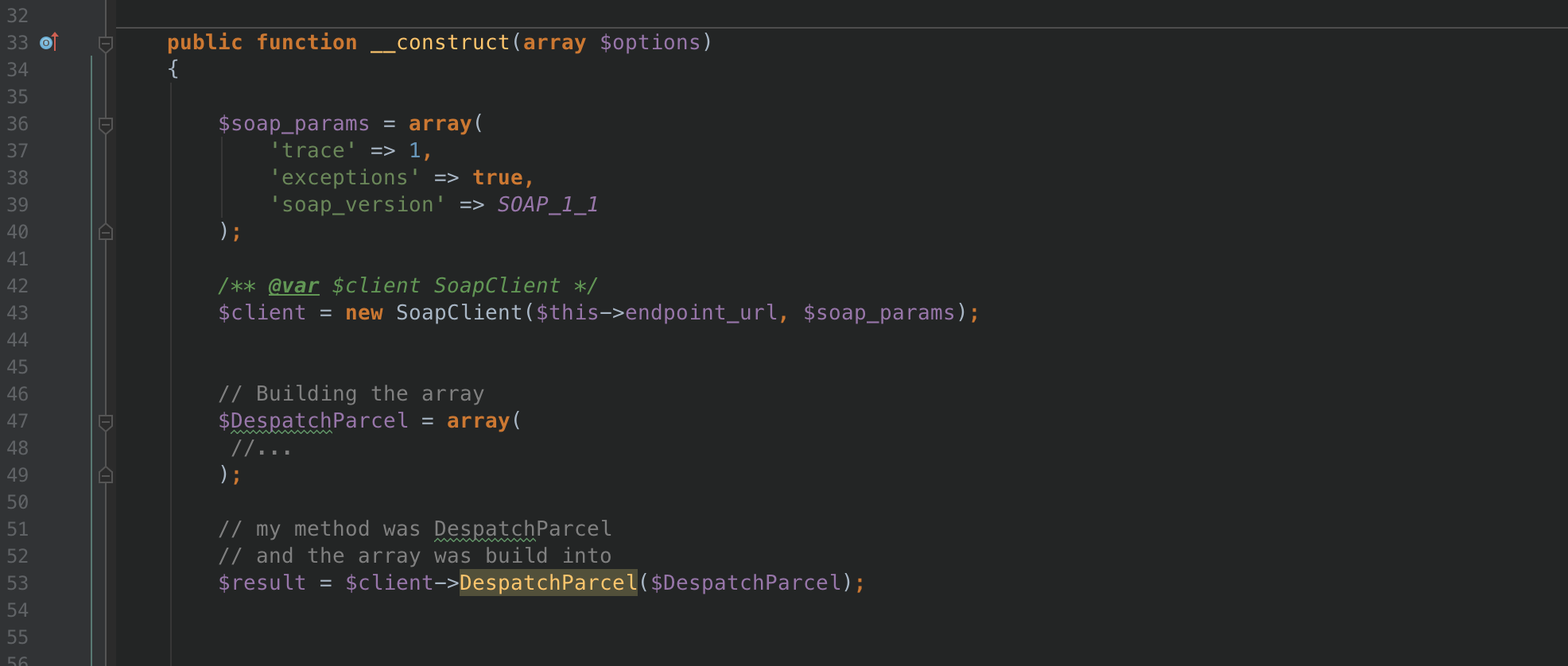
Troubleshooting SOAP: requesting multiple elements
I’m integrating the Whistl shipping carrier in our custom framework through SOAP protocol.
The fastest way is to build an array with all the required elements and send it to Whistl API.
Working with SOAP in php is very simple. Especially when you use the array as a container to pass to SOAP.
Example of SOAP initialisation and the method to be called with the array:
// initiate the soap request $client = new SoapClient($url, array("soap_version" => SOAP_1_1,"trace" => 1)); // Building the array $DespatchParcel = array( ... ); // my method was DespatchParcel // and the array was build into $result = $client->DespatchParcel($DespatchParcel);
But later, going a bit closer through documentation spotted this bit of requirement for the request:
<ifor1:ParcelProducts> <ifor1:parcelProduct> <ifor1:Code>30205958</ifor1:Code> <ifor1:Quantity>1</ifor1:Quantity> </ifor1:parcelProduct> <ifor1:parcelProduct> <ifor1:Code>30205959</ifor1:Code> <ifor1:Quantity>2</ifor1:Quantity> </ifor1:parcelProduct> </ifor1:ParcelProducts>
And at that moment I realised, there’s no way I could pass multiple elements with the same tag (<parcelProduct>
) using the array.
Overcome the multiple elements restriction
Tried multiple approaches and the best solution (the one and only one) was to use the empty generic php Object.
It allows you to pass a request with same multiple elements, for example the upper XML request
would look like:
// new object $DespatchParcel = new stdClass(); $parcelProduct1 = new stdClass(); // assign property $parcelProduct1->Code = "30205958"; $parcelProduct1->Quantity = "1"; $parcelProduct2 = new stdClass(); $parcelProduct2->Code = "30205959"; $parcelProduct2->Quantity = "2"; $ParcelProducts = new stdClass(); $ParcelProducts->parcelProduct = $parcelProduct1; $ParcelProducts->parcelProduct = $parcelProduct2; $DespatchParcel->ParcelProducts = $ParcelProducts;
After the object is properly build and is passed to the method as in the following example:
// Make the SOAP call $result = $client->DespatchParcel($DespatchParcel);
That’s what I’m calling a neat solution
😀 !
P.S. Don’t forget to pass every bit of code through GIT, that’s a life saver.